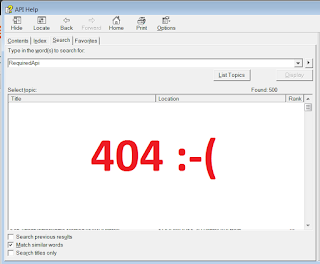
SOLIDWORKS API is a powerful toolset covering majority of SOLIDWORKS functions. However in some cases certain APIs are not available. This could potentially put the whole development project at risk as workaround is not always possible.
In this post I will discuss alternative solutions of calling SOLIDWORKS commands when API cannot be used (either not available or faulty).
Running commands from swCommands_e enumeration
SOLIDWORKS provides more than 3000 commands identifications via swCommands_e enumeration which can be invoked via ISldWorks::RunCommand method.
Follow the Capture SOLIDWORKS Commands article for more information and macro to simplify capturing of the ids of invoked commands.
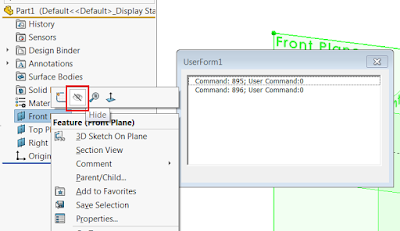
Refer the Display Assembly Visualization Page for a code example for invoking the command.
These commands are usually used to invoke dialog buttons (including Property Manager Page) or toolbar/menu buttons which cannot be called from the core SOLIDWORKS API.
By unknown reasons all the commands invoked via ISldWorks::RunCommand method introduce about 1 second delay. If multiple commands need to be invoked this may cause the performance issue. Refer the next section for an alternative solution.
Calling Windows Command
Although swCommands_e enumeration described above is very powerful, there are still may be some commands unavailable.
I will describe another way of calling the commands using Windows API.
SOLIDWORKS is Win32 application which means that all the communication is routed via message map. Those commands can be called from the Windows API functions, such as SendMessage.
The challenge is how to discover the id of the required command.
Fortunately, multiple tools available allowing to hook Win32 messages from Windows application. One of these tools is Spy++ built into MS Visual Studio.
This tool is available from the TOOLS menu in MS Visual Studio IDE.
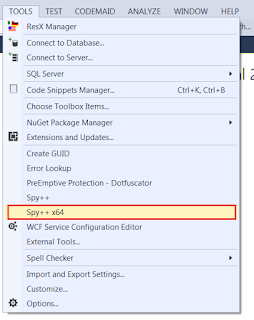
I would recommend to set the log options to only capture WM_COMMAND message.
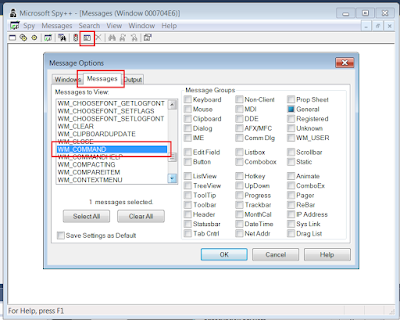
Simply find the SOLIDWORKS Window, click required command and capture the wParam value
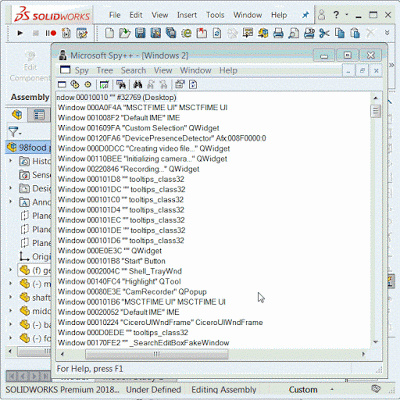
The value recorded as wParam is a command id. Use the following code to invoke this command.
#If VBA7 Then
Private Declare PtrSafe Function SendMessage Lib "User32" Alias "SendMessageA" (ByVal hWnd As Long, ByVal wMsg As Long, ByVal wParam As Long, lParam As Any) As Long
#Else
Private Declare Function SendMessage Lib "User32" Alias "SendMessageA" (ByVal hWnd As Long, ByVal wMsg As Long, ByVal wParam As Long, lParam As Any) As Long
#End If
Dim swApp As SldWorks.SldWorks
Sub main()
Set swApp = Application.SldWorks
RunWmCommand swApp, 33227
End Sub
Sub RunWmCommand(swApp As SldWorks.SldWorks, cmd As Long)
Const WM_COMMAND As Long = &H111
Dim swFrame As SldWorks.Frame
Set swFrame = swApp.Frame
SendMessage swFrame.GetHWnd(), WM_COMMAND, cmd, 0
End Sub
Examples
Sending message in unexpected way (e.g. calling the hide sketch where document is not opened and sketch is not selected) might result in unpredictable behavior (including crash). Always check the conditions before sending Win32 message.