eDrawings is a free SOLIDWORKS file viewer. Software can be downloaded from the eDrawingsViewer web-site. This application not only allows to view, markup, measure and print SOLIDWORKS, DXF and DWG files without having SOLIDWORKS installed, but also provides API for a programmatic access to its features.
In this blog article I will guide you though the basics of eDrawings API. I will show how to host the eDrawings control on Windows Forms or WPF application. Introduce to the eDrawings markup API to extract the measurements. And finally describe 3 most common use cases where eDrawings can be useful for, including the application to batch export SOLIDWORKS drawing to PDF format without having SOLIDWORKS installed or even without a need to own a SOLIDWORKS license.
Creating Windows Forms application
eDrawings interop provides signatures of API methods which can be used when programming in C# or VB.NET and located in the installation folder of eDrawings: %commonprogramfiles%\eDrawings[Version]\eDrawings.Interop.EModelViewControl.dll.
Simply create a wrapper around eDrawings ActiveX control and add it to your form. Now you can explore eDrawings API!
public class EDrawingHost : AxHost
{
public EDrawingHost() : base("22945A69-1191-4DCF-9E6F-409BDE94D101")
{
m_IsLoaded = false;
}
}
Follow the Hosting eDrawings control in Windows Forms for detailed instruction and code examples.
Creating WPF application
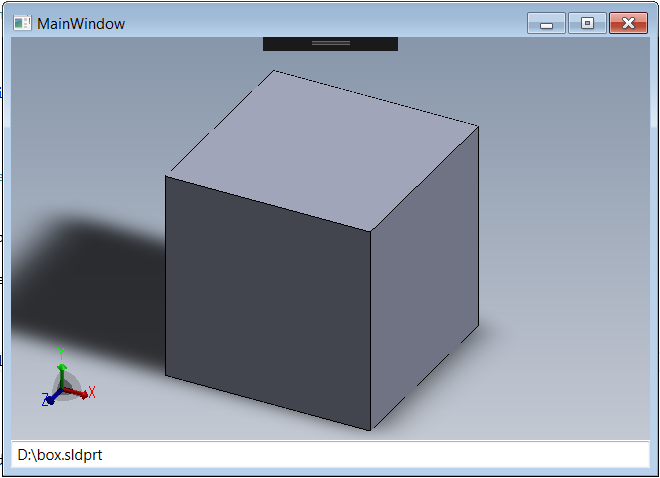
If you are using the modern Windows Presentation Foundation (WPF) framework and want to host your eDrawings control in there you can do it by wrapping your control in the WindowsFormsHost
public partial class eDrawingsHostControl : UserControl
{
private EModelViewControl m_Ctrl;
public eDrawingsHostControl()
{
InitializeComponent();
var host = new WindowsFormsHost();
var ctrl = new EDrawingHost();
host.Child = ctrl;
this.AddChild(host);
}
}
Follow the Hosting SOLIDWORKS eDrawings control in Windows Presentation Foundation (WPF) for detailed instruction and code examples.
Markup
One of the cool eDrawings features is an ability to add markups to the files. This includes stamps, comments, lines, arcs, clouds etc. It is also possible to perform measurements directly in the eDrawings viewer. Markup API is available in IEModelMarkupControl interface.
Follow Utilizing markup functionality using SOLIDWORKS eDrawings API tutorial for a guide and project example of using eDrawings markup API.
Use Cases
Although eDrawings API is relatively small compared to SOLIDWORKS API, it is still very powerful and can be used in the number of applications. Below are the most common scenarios of utilizing eDrawings API
Batch export to pdf
There is no way to export eDrawings files into foreign format, however the file can be printed and if PDF printer is setup, eDrawings can be used to effectively batch convert drawings into a PDF format. You can find the application which is doing this by following: Batch export SOLIDWORKS files to PDF via eDrawings API (without SOLIDWORKS) link.
File viewer
This would be probably the most common use of eDrawings. If you are building Product Data Management or Product Catalogue applications and want to enable viewing of files eDrawings would provide simple and lightweight way to do this.
Inspection tool
Using eDrawings markup capabilities it is possible to implement the inspection tool where users will be able to add markups, review comments and stamps to the files in the business workflow.