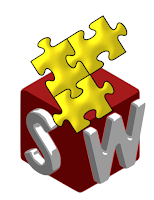
SOLIDWORKS add-ins are great options for automating SOLIDWORKS. But what if the add-in itself needs to be automated? This enables a whole new functionality as now add-in functions can be called from macros, add-ins, stand-alone applications or even scripts. So effectively the add-in is exposing API itself.
There are multiple ways to add API to your add-in. In this blog post we will take a look in automating add-ins via COM interface and ISldWorks::GetAddInObject SOLIDWORKS API method.
When developing add-ins with .NET language (C# or VB.NET) it is required to enable COM communication via COM visible interfaces:
- Create public interface which exposes the API functions required to be accessed by external applications. Make this interface COM visible
- Implement this interface in your main add-in class
- Register assembly for COM interops
[ComVisible(true)]
public interface IMyAddInApi
{
double DoSomething(int someParam);
}
[ComVisible(true), Guid("799A191E-A4CF-4622-9E77-EA1A9EF07621")]
public class MyAddIn : ISwAddIn
{
...
public double DoSomething(int someParam)
{
//Implement
}
}
As the result type library (*.tlb) file is generated. This now can be referenced in the macro or stand-alone application and add-in API can be called.
Dim swApp As SldWorks.SldWorks
Sub main()
Set swApp = Application.SldWorks
Dim swMyAddIn As CodeStack.IMyAddInApi
Set swMyAddIn = swApp.GetAddInObject("CodeStack.MyAddInApi")
Dim res As Double
res = swMyAddIn.DoSomething(10)
End Sub
Refer the Call function of SOLIDWORKS add-in object from stand-alone application or macro for detailed guide and code examples.